1.合并两个链表
将两个升序链表合并为一个新的 升序
链表并返回。新链表是通过拼接给定的两个链表的所有节点组成的。
屏幕截图 2022-01-20 150104
示例 1:
输入:l1 = [1,2,4], l2 = [1,3,4] 输出:[1,1,2,3,4,4]
暴力法:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
|
struct ListNode* mergeTwoLists(struct ListNode* list1, struct ListNode* list2){ struct ListNode* HeadNode=(struct ListNode* )malloc(sizeof(struct ListNode)); struct ListNode* pMove=HeadNode; while(list1&&list2){ if(list1->val<=list2->val){ pMove->next=list1; list1=list1->next; }else{ pMove->next=list2; list2=list2->next; } pMove=pMove->next; } if(list1) pMove->next=list1; else pMove->next=list2; return HeadNode->next; }
|
2.逆转链表
给你单链表的头节点 head
,请你反转链表,并返回反转后的链表。
> 输入:head = [1,2] > 输出:[2,1]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
|
struct ListNode* reverseList(struct ListNode* head){ struct ListNode* pre=NULL; struct ListNode* cur=head; while(cur) { struct ListNode* next=cur->next; cur->next=pre; pre=cur; cur=next; } return pre; }
|
以下是动图: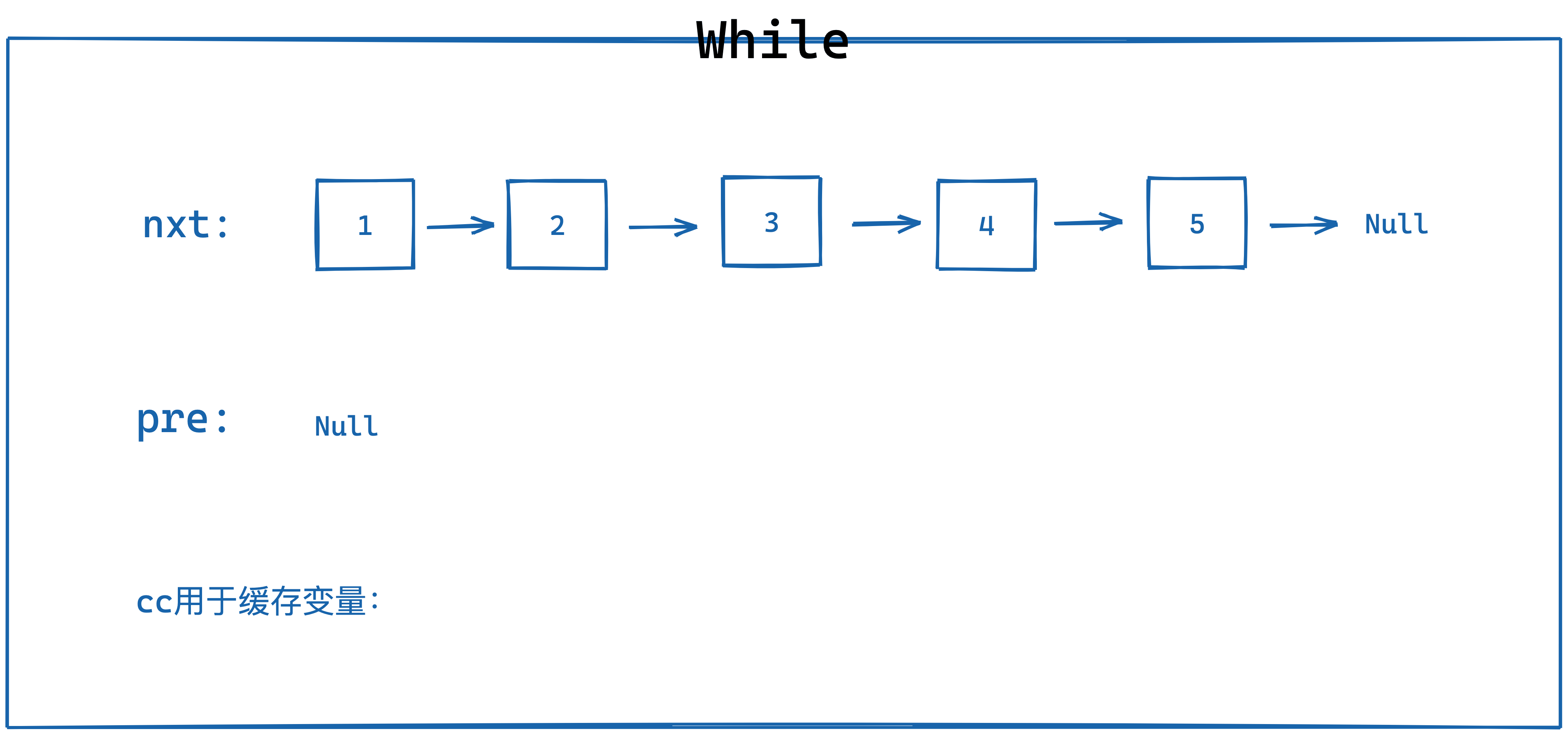
3. 删除链表中重复的节点
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| 在一个排序的链表中,存在重复的节点,请删除该链表中重复的节点,重复的节点不保留。
数据范围 链表中节点 val 值取值范围 [0,100]。 链表长度 [0,100]。
样例1 输入:1->2->3->3->4->4->5
输出:1->2->5 样例2 输入:1->1->1->2->3
输出:2->3
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
|
struct ListNode* deleteDuplication(struct ListNode* head) { struct ListNode* pHead=(struct ListNode*)malloc(sizeof(struct ListNode)); pHead->next=head; struct ListNode* pre=pHead; struct ListNode* cur=head; if(!head) return; while(cur) { while(cur&&pre->next->val==cur->val) cur=cur->next; if(pre->next->next==cur) pre=pre->next; else pre->next=cur; } return pHead->next; }
|
warning:不能采用pre和cur都是首节点,然后开始判断的情况,因为会有这种特殊情况:从首节点开始到尾就是重复了